iOS¶
Here are the specific properties you can configure for the iOS output:
- Declaring iOS libraries
- Bundles
- Bitcode
- Strip Swift Symbols
- PList
- Entitlements
- Pods
- Run Script (Xcode)
- Resource files
Declaring iOS libraries¶
Please refer to the dedicated documentation to learn how to add external iOS libraries.
Bundles¶
Additionaly to libraries, you may want to add resources thanks to bundles in your iOS application. To have more information about bundle, please look at the Apple documentation on the subject.
<urs>
[...]
<specific>
<ios>
<bundle path="path/to/mybundle.bundle"/>
</ios>
</specific>
</urs>
Path to bundles can be relative to the project or absolute.
To know if the bundle was well inserted in the iOS output project, the generated Xcode project can be opened.
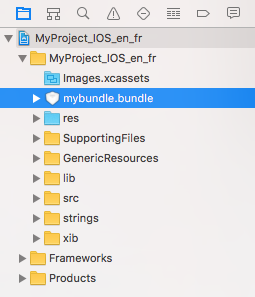
Bitcode¶
By default, NeoMAD does not generate the bitcode for the iOS target. If you
need to generate it, it can be done with the <enableBitcode>
URS tag.
<urs>
[...]
<specific>
<ios>
<enableBitcode>true</enableBitcode>
</ios>
</specific>
</urs>
You can check the result of the property insertion in the generated Xcode project.
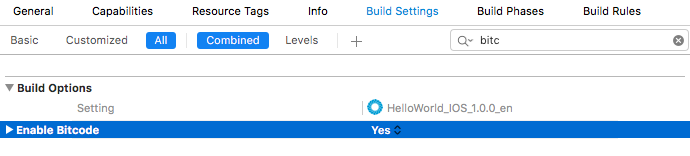
Strip Swift Symbols¶
Strip Swift Symbols iOS build property can be activated or deactivated form the URS
using <stripSwiftSymbols>
tag.
<urs>
[...]
<specific>
<ios>
<stripSwiftSymbols>true</stripSwiftSymbols>
</ios>
</specific>
</urs>
PList¶
NeoMAD generates a default plist for the application. However, you may need to add specific information
for your application in the plist. To do this, simply add the plist extra content directly in the URS
in the <plist>
tag.
<urs>
[...]
<specific>
<ios>
<plist>
<key>Array</key>
<array>
<string>value1</string>
<string>value2</string>
</array>
<key>Boolean</key>
<true/>
</plist>
</ios>
</specific>
</urs>
You can check that the plist properties were inserted by opening the generated Xcode project.
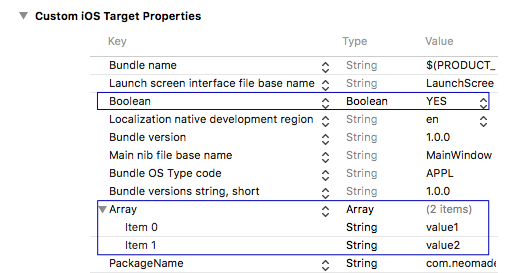
Entitlements¶
You may need to add entitlements for your iOS version of the application to use some specific API.
To do so in NeoMAD, you can use the <entitlements>
tag.
<urs>
[...]
<specific>
<ios>
<entitlements>
<key>keychain-access-groups</key>
<array>
<string>$(AppIdentifierPrefix)com.microsoft.intune.mam</string>
<string>$(AppIdentifierPrefix)com.microsoft.adalcache</string>
</array>
</entitlements>
</ios>
</specific>
</urs>
Pods¶
Pods can be added in project to use in specific code. Pods must be declared in the URS:
<urs>
[...]
<specific>
<ios>
<podfile minTargetVersion="10.0">
<property>use_frameworks!</property>
<property isGlobal="true">source 'https://github.com/CocoaPods/Specs.git'</property>
<pod name="OneSignal" version="2.14.3"/>
</podfile>
</ios>
</specific>
</urs>
- <podfile minTargetVersion=”10.0”>: main entry of the podfile section where minTargetVersion is the minimum iOS version supported by the project.
- <property isGlobal=”true”>use_frameworks!</property>: a property (lines) to add to the PodFile. By default, it is added to the target in the Podfile. Use isGlobal to apply it to all the Podfile.
- <pod name=”OneSignal” />: a pod to add to the project. version, git, commit, branch, tag or path can be set as attribut depending of the origin of the pod
As an example, the previous pod declaration will generate the following Podfile (target name is automatically set by NeoMAD)
platform :ios, '10.0'
source 'https://github.com/CocoaPods/Specs.git'
target 'MyTarget' do
use_frameworks!
pod 'OneSignal', '2.14.3'
end
NeoMAD will download and install the pods at the project generation steps using the CocoaPods command configured on the Mac.
Some optional properties may be added to the podfile section if more customizations are required:
- <postinstall>: will add the content of the section in the Podfile in a post_install section. For more convenience, ‘n’ must be add at the end of lines in the URS section if line breaks are required in the output Podfile.
<urs>
[...]
<specific>
<ios>
<podfile minTargetVersion="10.0">
<postinstall>
installer.aggregate_targets.each do |target|\n
target.xcconfigs.each do |variant, xcconfig|\n
xcconfig_path = target.client_root + target.xcconfig_relative_path(variant)\n
IO.write(xcconfig_path, IO.read(xcconfig_path).gsub("DT_TOOLCHAIN_DIR", "TOOLCHAIN_DIR"))\n
end\n
end\n
</postinstall>
</podfile>
</ios>
</specific>
</urs>
Run Script (Xcode)¶
Sometimes to use some libraries or pods, additional Run Script Build Phase
may be required when building with Xcode.
Run Script Build Phase
can be added with NeoMAD by the URS. The keys are the same as for an Xcode project.
<urs>
[...]
<specific>
<ios>
<runScriptPhase name="Script name">
<shellScript>shell/command</shellScript>
<shellPath>/bin/sh</shellPath>
<dependencyFile>path/to/dependency/file</dependencyFile>
<inputPaths>
<path>path/to/input/path/1</path>
<path>path/to/input/path/2</path>
</inputPaths>
<inputFileListPaths>
<path>path/to/input/inputFileListPaths</path>
</inputFileListPaths>
<outputPaths>
<path>path/to/output/path/1</path>
</outputPaths>
<outputFileListPaths>
<path>path/to/output/outputFileListPaths</path>
</outputFileListPaths>
<showEnvVarsInLog>false</showEnvVarsInLog>
<runOnlyForDeploymentPostProcessing>false</runOnlyForDeploymentPostProcessing>
</runScriptPhase>
</ios>
</specific>
</urs>
Resource files¶
Sometimes to use some libraries or pods, specific configuration files should be included in Xcode. They are not classical NeoMAD resources files. They are just files to add in the Xcode project.
Such files can be added via the urs <specific><ios><file>
tag.
<urs>
[...]
<specific>
<ios>
<file path="path/to/my/resource.file" group="ProjectGroup" build="false"/>
</ios>
</specific>
</urs>
path
(mandatory): path to the file in the input projectgroup
(optional): group where the file must be copied in the Xcode project. If the group does not exist, it will be created. Default is the root group of the project.build
(optional): does the file need to be added to the build files of the project (default false).