Specifying keyboard options¶
Virtual keyboards can be customized to help users input text in the application. This document presents some options that can be used to facilitate text input.
Text mode¶
Sometimes an application expects the user to type a certain class of text, e.g. an email or a number. To ease user input, the developer can choose to present an adapted keyboard. In some cases the text mode is just a hint. The user can still change the kind of keyboard displayed, so the developer should always check that the typed text is valid. By default the text mode is configured to show the keyboard to type any raw text.
The following chapters detail the available text modes, how to set them and their appearance on each platform.
ANY text mode¶
This mode will make the default keyboard appear, in which the user can type any alphanumeric character.
The mode can be set in XML layouts:
<TextField textMode="ANY" />
The same result can be obtained with Java code:
myTextField.setTextMode(TextMode.ANY);
The following screenshots show this keyboard appearance on each platform:
![]() |
![]() |
iOS ANY keyboard | Android ANY keyboard |
EMAIL_ADDRESS text mode¶
This mode will show a keyboard that ease the typing of an email address, for example with the ‘@’ character.
The mode can be set in XML layouts:
<TextField textMode="EMAIL_ADDRESS" />
The same result can be obtained with Java code:
myTextField.setTextMode(TextMode.EMAIL_ADDRESS);
The following screenshots show this keyboard appearance on each platform:
![]() |
![]() |
iOS EMAIL_ADDRESS keyboard | Android EMAIL_ADDRESS keyboard |
NUMERIC text mode¶
This mode will show a keyboard that ease the typing of numbers.
The mode can be set in XML layouts:
<TextField textMode="NUMERIC" />
The same result can be obtained with Java code:
myTextField.setTextMode(TextMode.NUMERIC);
The following screenshots show this keyboard appearance on each platform:
![]() |
![]() |
iOS NUMERIC keyboard | Android NUMERIC keyboard |
PHONE_NUMBER keyboard appearance¶
This mode will show a keyboard that ease the typing of phone numbers.
The mode can be set in XML layouts:
<TextField textMode="PHONE_NUMBER" />
The same result can be obtained with Java code:
myTextField.setTextMode(TextMode.PHONE_NUMBER);
The following screenshots show this keyboard appearance on each platform:
![]() |
![]() |
iOS PHONE_NUMBER keyboard | Android PHONE_NUMBER keyboard |
Auto-correcting text¶
When raw text needs to be typed by the user, the developer can choose to help him by setting the auto-correct option for text fields. This way if the user makes a spelling mistake on a word, the text will be automatically corrected. By default the auto-correct option is activated on any TextField and TextArea.
This option can be set in XML layouts:
<TextField autoCorrect="true" />
The same result can be obtained with Java code:
myTextField.setAutoCorrect(true);
Limitations¶
Cross-platform¶
The auto-correct option is only supported on Android and iOS platforms.
Auto-correct and suggestions¶
When the auto-correct option is enabled, a list of suggestions generally appears above the keyboard, to help the user correct a word. The suggestions can not be hidden for the moment. When the auto-correct option is disabled, the display of suggestions is platform-dependant.
Here is what looks like the suggestions list on an Android keyboard :
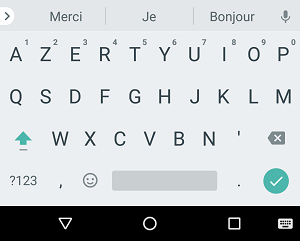
System settings¶
On several platforms, the auto-correct option can be set directly in the System settings. The System setting is in general prioritary on the option set on each individual text field.
Capitalize mode¶
Sometimes an application expects the user to type text in a certain form, e.g. for a person full name each word should start with a capital. To help the user type this kind of text, the developer can choose to help him by setting an appropriate capitalize mode for text fields. The capitalize mode does not enforce users to use the specified case, it is just a hint. The user can still change the mode explicitly on the keyboard. By default only the first letter of a sentence is capitalized.
SENTENCES capitalize mode¶
This mode capitalizes the first letter of each sentence. It is the default mode.
The mode can be set in XML layouts:
<TextField capitalizeMode="SENTENCES" />
The same result can be obtained with Java code:
myTextField.setCapitalizeMode(CapitalizeMode.SENTENCES);
If the user types text without changing the mode manually, typed text will look like:
This is the first sentence. This is the second sentence.
WORDS capitalize mode¶
This mode capitalizes the first letter of each word.
The mode can be set in XML layouts:
<TextField capitalizeMode="WORDS" />
The same result can be obtained with Java code:
myTextField.setCapitalizeMode(CapitalizeMode.WORDS);
If the user types text without changing the mode manually, typed text will look like:
This Is The First Sentence. This Is The Second Sentence.
CHARACTERS capitalize mode¶
This mode capitalizes each character of the typed text.
The mode can be set in XML layouts:
<TextField capitalizeMode="CHARACTERS" />
The same result can be obtained with Java code:
myTextField.setCapitalizeMode(CapitalizeMode.CHARACTERS);
If the user types text without changing the mode manually, typed text will look like:
THIS IS THE FIRST SENTENCE. THIS IS THE SECOND SENTENCE.
NONE capitalize mode¶
This mode does not capitalize any character. All characters will be lower case.
The mode can be set in XML layouts:
<TextField capitalizeMode="NONE" />
The same result can be obtained with Java code:
myTextField.setCapitalizeMode(CapitalizeMode.NONE);
If the user types text without changing the mode manually, typed text will look like:
this is the first sentence. this is the second sentence.